6.1. Memento¶
EN: Memento
PL: Pamiątka
Type: object
The Memento design pattern is a behavioral design pattern that allows an object to save and restore its previous state. This is useful when you need to provide some sort of undo functionality in your application.
In Python, we can implement the Memento pattern using classes. Here's a simple example:
First, we define a Memento
class that will hold the state of the
Originator
:
>>> class Memento:
... def __init__(self, state):
... self._state = state
...
... def get_saved_state(self):
... return self._state
Then, we define an Originator
class that has a state and creates a memento
object to save its state:
>>> class Originator:
... _state = ""
...
... def set(self, state):
... print(f"Originator: Setting state to {state}")
... self._state = state
...
... def save_to_memento(self):
... print("Originator: Saving to Memento.")
... return Memento(self._state)
...
... def restore_from_memento(self, memento):
... self._state = memento.get_saved_state()
... print(f"Originator: State after restoring from Memento: {self._state}")
Next, we define a Caretaker
class that keeps track of multiple mementos:
>>> class Caretaker:
... def __init__(self):
... self._saved_states = []
...
... def add_memento(self, memento):
... self._saved_states.append(memento)
...
... def get_memento(self, index):
... return self._saved_states[index]
Finally, we can use the Originator
, Memento
, and Caretaker
classes
like this:
>>> caretaker = Caretaker()
>>> originator = Originator()
>>>
>>> originator.set("State1")
Originator: Setting state to State1
>>> originator.set("State2")
Originator: Setting state to State2
>>> caretaker.add_memento(originator.save_to_memento())
Originator: Saving to Memento.
>>>
>>> originator.set("State3")
Originator: Setting state to State3
>>> caretaker.add_memento(originator.save_to_memento())
Originator: Saving to Memento.
>>>
>>> originator.set("State4")
Originator: Setting state to State4
>>> originator.restore_from_memento(caretaker.get_memento(0))
Originator: State after restoring from Memento: State2
>>> originator.restore_from_memento(caretaker.get_memento(1))
Originator: State after restoring from Memento: State3
In this example, the Originator
sets and changes its state. It can also
create a Memento
that represents its current state. The Caretaker
can
keep track of these mementos and restore the Originator
to its previous
states.
6.1.1. Pattern¶
Undo operation
Remembering state of objects
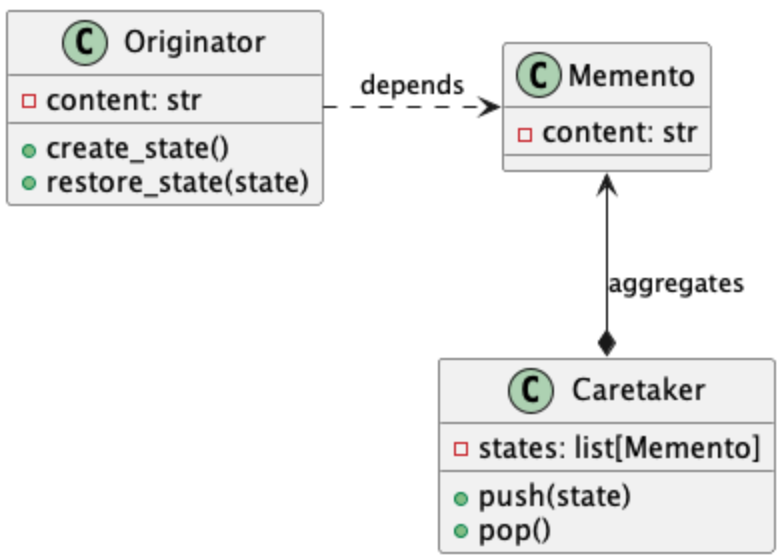
```plantuml
class Originator {
- content: str
+ create_state()
+ restore_state(state)
}
class Memento {
- content: str
}
class Caretaker {
- states: list[Memento]
+ push(state)
+ pop()
}
Memento -down[plain]-* Caretaker : composition
Originator -right[dashed]-> Memento : dependency
```
6.1.2. Problem¶

```plantuml
class Editor {
- content: str
+ create_state()
+ restore_state(state)
}
class EditorState {
- content: str
}
class History {
- states: list[EditorState]
+ push(state)
+ pop()
}
EditorState -down[plain]-* History : composition
Editor -right[dashed]-> EditorState : dependency
```
6.1.3. Solution¶
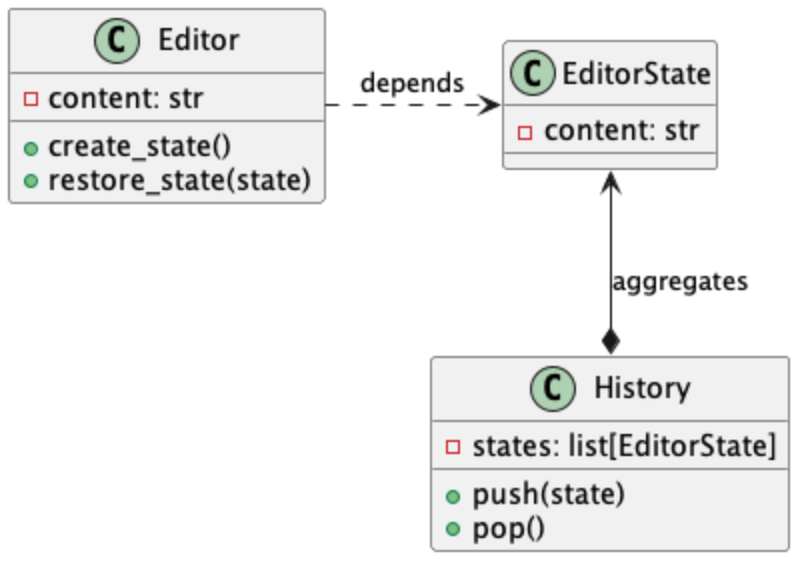
from dataclasses import dataclass, field
@dataclass(frozen=True)
class EditorState:
content: str
@dataclass
class History:
states: list[EditorState] = field(default_factory=list)
def push(self, state: EditorState) -> None:
self.states.append(state)
def pop(self) -> EditorState:
return self.states.pop()
class Editor:
content: str
def set_content(self, content: str) -> None:
self.content = content
def get_content(self) -> str:
return self.content
def create_state(self):
return EditorState(self.content)
def restore_state(self, state: EditorState):
self.content = state.content
if __name__ == '__main__':
editor = Editor()
history = History()
editor.set_content('a')
print(editor.content)
# a
editor.set_content('b')
history.push(editor.create_state())
print(editor.content)
# b
editor.set_content('c')
print(editor.content)
# c
editor.restore_state(history.pop())
print(editor.content)
# b
6.1.4. Assignments¶
"""
* Assignment: DesignPatterns Behavioral Memento
* Complexity: medium
* Lines of code: 29 lines
* Time: 13 min
English:
1. Implement Memento pattern
2. Create account history of transactions with:
a. `when: datetime` - date and time of a transaction
b. `amount: float` - transaction amount
3. Allow for transaction undo
4. Run doctests - all must succeed
Polish:
1. Zaimplementuj wzorzec Memento
2. Stwórz historię transakcji na koncie z:
a. `when: datetime` - data i czas transakcji
b: `amount: float` - kwota transakcji
3. Pozwól na wycofywanie (undo) transakcji
4. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> account = Account()
>>> account.deposit(100.00)
>>> account.balance
100.0
>>> account.deposit(50.00)
>>> account.balance
150.0
>>> account.deposit(25.00)
>>> account.balance
175.0
>>> account.undo()
>>> account.balance
150.0
"""
from dataclasses import dataclass, field
from datetime import datetime
from typing import Final
@dataclass
class Account:
balance: float = 0.0
def deposit(self, amount: float) -> None:
raise NotImplementedError
def undo(self):
raise NotImplementedError