5.7. DataFrame At
.at[row, col]
- fancy indexing.iat[row, col]
- integer at (no fancy indexing)Access a single value for a row/column pair by integer position
Use iat if you need to get or set a single value in a DataFrame or Series
Pandas Select Cell:
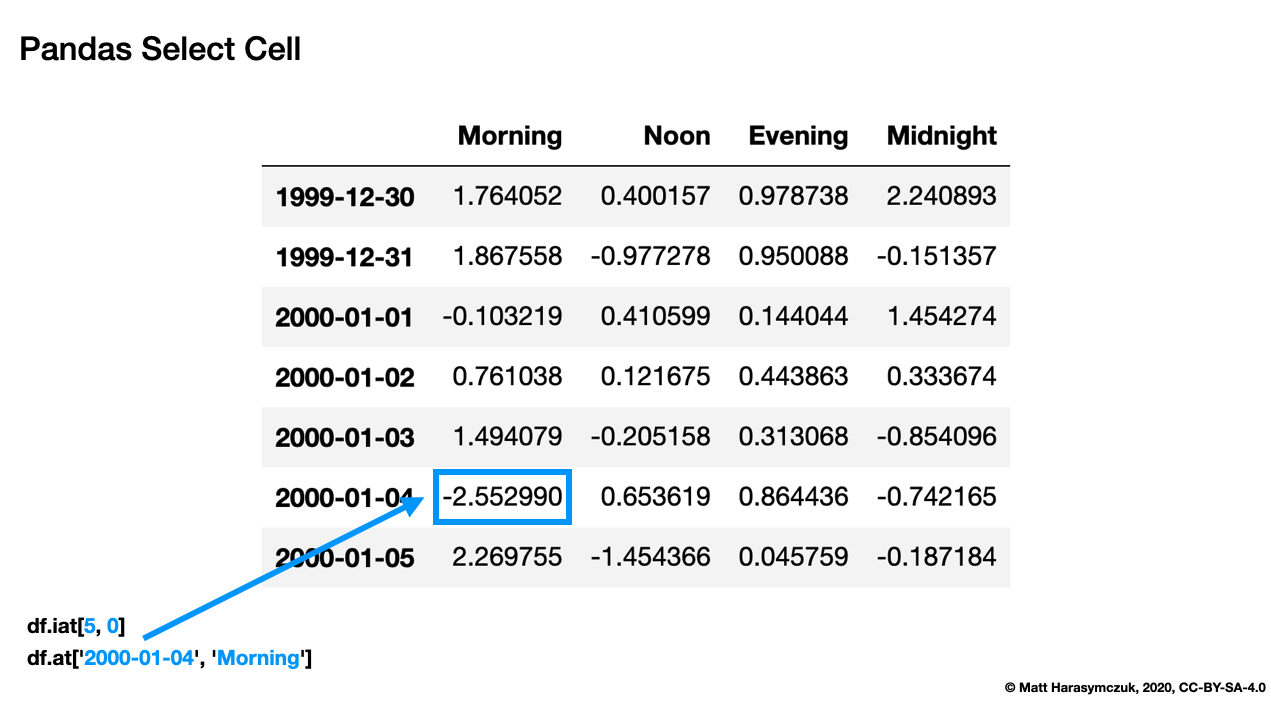
5.7.1. SetUp
>>> import pandas as pd
>>> import numpy as np
>>> np.random.seed(0)
>>>
>>>
>>> df = pd.DataFrame(
... columns = ['Morning', 'Noon', 'Evening', 'Midnight'],
... index = pd.date_range('1999-12-30', periods=7),
... data = np.random.randn(7, 4))
>>>
>>> df
Morning Noon Evening Midnight
1999-12-30 1.764052 0.400157 0.978738 2.240893
1999-12-31 1.867558 -0.977278 0.950088 -0.151357
2000-01-01 -0.103219 0.410599 0.144044 1.454274
2000-01-02 0.761038 0.121675 0.443863 0.333674
2000-01-03 1.494079 -0.205158 0.313068 -0.854096
2000-01-04 -2.552990 0.653619 0.864436 -0.742165
2000-01-05 2.269755 -1.454366 0.045759 -0.187184
5.7.2. Get value at specified row/column pair
First argument is column
Second argument is row
>>> df.iat[0,0]
np.float64(1.764052345967664)
>>>
>>> df.iat[1,0]
np.float64(1.8675579901499675)
>>>
>>> df.iat[0,1]
np.float64(0.4001572083672233)
5.7.3. Get value from row
loc
returns Series
>>> df.loc['2000-01-01'].iat[1]
np.float64(0.41059850193837233)
5.7.4. Set value at a position
>>> df.iat[0,0] = pd.NA
>>> df
Morning Noon Evening Midnight
1999-12-30 NaN 0.400157 0.978738 2.240893
1999-12-31 1.867558 -0.977278 0.950088 -0.151357
2000-01-01 -0.103219 0.410599 0.144044 1.454274
2000-01-02 0.761038 0.121675 0.443863 0.333674
2000-01-03 1.494079 -0.205158 0.313068 -0.854096
2000-01-04 -2.552990 0.653619 0.864436 -0.742165
2000-01-05 2.269755 -1.454366 0.045759 -0.187184