7.1. Mapping Dict
dict
are key-value storage (HashMap)Mutable - can add, remove, and modify items
Since Python 3.7:
dict
keeps order of elementsBefore Python 3.7:
dict
order is not ensured!!
How are dictionaries implemented in CPython? [1]
CPython's dictionaries are implemented as resizable hash tables. Compared to B-trees, this gives better performance for lookup (the most common operation by far) under most circumstances, and the implementation is simpler.
Dictionaries work by computing a hash code for each key stored in the
dictionary using the hash()
built-in function. The hash code varies
widely depending on the key and a per-process seed; for example, "Python"
could hash to -539294296 while "python", a string that differs by a single
bit, could hash to 1142331976. The hash code is then used to calculate
a location in an internal array where the value will be stored. Assuming
that you're storing keys that all have different hash values, this means
that dictionaries take constant time – O(1), in Big-O notation – to retrieve
a key.
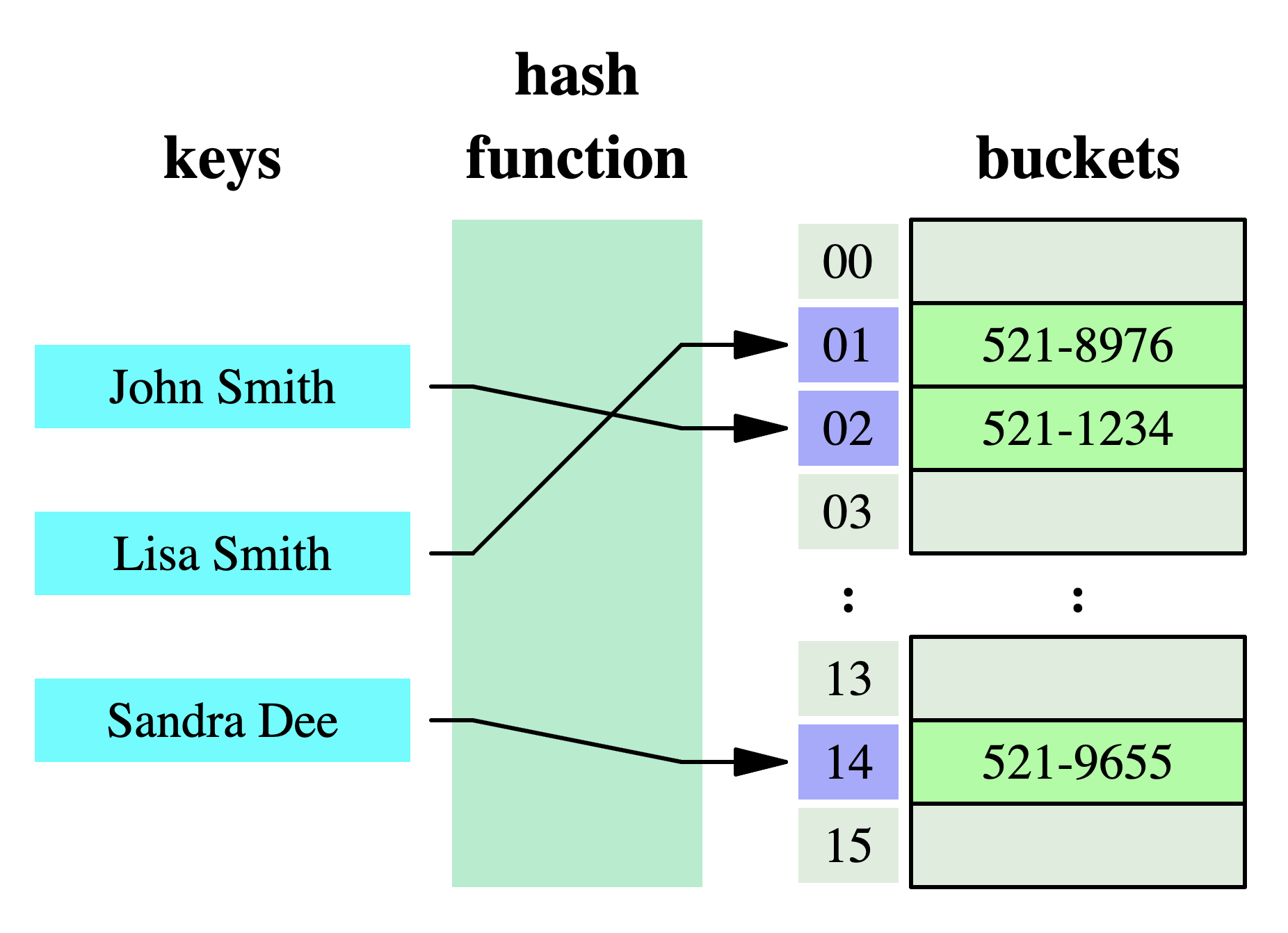
7.1.1. Define Empty
{}
is fasterdict()
is more verbose
>>> data = {}
>>> data = dict()
7.1.2. Define With Elements
Comma after last element is optional
>>> data = {
... 'firstname': 'Mark',
... 'lastname': 'Watney',
... 'age': 41,
... }
>>> data = dict(
... firstname='Mark',
... lastname='Watney',
... age=41,
... )
7.1.3. Duplicates
Duplicating items are overridden by latter:
>>> data = {
... 'firstname': 'Mark',
... 'firstname': 'Melissa',
... }
>>>
>>> data
{'firstname': 'Melissa'}
7.1.4. Dict vs. Set
Both
set
anddict
keys must be hashableBoth
set
anddict
uses the same{
and}
bracesDespite similar syntax, they are different types
Set:
>>> data = {'Mark', 'Melissa', 'Rick'}
Dict:
>>> data = {'Mark':None, 'Melissa':None, 'Rick':None}
set()
values behaves similar to dict()
keys.
This is one of the reasons why they both have the same brackets.
7.1.5. List of Pairs
Pair:
>>> data = ('firstname', 'Mark')
List of pairs:
>>> data = [
... ('firstname', 'Mark'),
... ('lastname', 'Watney'),
... ('age', 41),
... ]
7.1.6. Convert List of Pairs
Convert list
of pairs to dict
:
>>> data = [
... ('firstname', 'Mark'),
... ('lastname', 'Watney'),
... ('age', 41),
... ]
>>>
>>> dict(data)
{'firstname': 'Mark', 'lastname': 'Watney', 'age': 41}
7.1.7. Length
>>> data = {
... 'firstname': 'Mark',
... 'lastname': 'Watney',
... 'age': 41,
... }
>>>
>>>
>>> len(data)
3
7.1.8. Use Case - 1
>>> MONTHS = {
... 1: 'January',
... 2: 'February',
... 3: 'March',
... 4: 'April',
... 5: 'May',
... 6: 'June',
... 7: 'July',
... 8: 'August',
... 9: 'September',
... 10: 'October',
... 11: 'November',
... 12: 'December'
... }
7.1.9. Use Case - 2
GIT - version control system
>>> git = {
... 'ce16a8ce': 'commit/1',
... 'cae6b510': 'commit/2',
... '895444a6': 'commit/3',
... 'aef731b5': 'commit/4',
... '4a92bc79': 'branch/master',
... 'b3bbd85a': 'tag/v1.0',
... }
7.1.10. References
7.1.11. Assignments
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Mapping Dict Create
# - Difficulty: easy
# - Lines: 3
# - Minutes: 2
# %% English
# 1. Define `result: dict` with:
# - key `firstname` with value `Mark`
# - key `lastname` with value `Watney`
# - key `group` with value `users`
# 2. Run doctests - all must succeed
# %% Polish
# 1. Zdefiniuj `result: dict` z:
# - kluczem `firstname` o wartości `Mark`
# - kluczem `lastname` o wartości `Watney`
# - kluczem `group` o wartości `users`
# 2. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> assert type(result) is dict, \
'Variable `result` has invalid type, should be dict'
>>> assert 'firstname' in result.keys(), \
'Value `firstname` is not in the result keys'
>>> assert 'lastname' in result.keys(), \
'Value `lastname` is not in the result keys'
>>> assert 'group' in result.keys(), \
'Value `group` is not in the result keys'
>>> assert 'Mark' in result['firstname'], \
'Value `Mark` is not in the result values'
>>> assert 'Watney' in result['lastname'], \
'Value `Watney` is not in the result values'
>>> assert 'users' in result['group'], \
'Value `users` is not in the result values'
"""
# Define `result: dict` with:
# - key `firstname` with value `Mark`
# - key `lastname` with value `Watney`
# - key `group` with value `users`
# type: dict[str,str]
result = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Mapping Dict ListOfPairs
# - Difficulty: easy
# - Lines: 1
# - Minutes: 2
# %% English
# 1. Use `DATA: list[tuple]`
# 2. Define `result: dict` with `DATA` converted to `dict`
# 3. Run doctests - all must succeed
# %% Polish
# 1. Użyj `DATA: list[tuple]`
# 2. Zdefiniuj `result: dict` z przekonwertowanym `DATA` do `dict`
# 3. Uruchom doctesty - wszystkie muszą się powieść
# %% Hints
# - `dict()`
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert type(result) is dict, \
'Variable `result` has invalid type, should be dict'
>>> assert all(type(x) is str for x in result.keys()), \
'All dict keys should be str'
>>> assert 'firstname' in result.keys()
>>> assert 'lastname' in result.keys()
>>> assert 'group' in result.keys()
>>> assert 'Mark' in result.values()
>>> assert 'Watney' in result.values()
>>> assert 'users' in result.values()
>>> pprint(result, width=40, sort_dicts=False)
{'firstname': 'Mark',
'lastname': 'Watney',
'group': 'users'}
"""
DATA = [
('firstname', 'Mark'),
('lastname', 'Watney'),
('group', 'users'),
]
# Define `result: dict` with `DATA` converted to `dict`
# type: dict[str,str]
result = ...