3.2. Numeric Float
Represents floating point number (vide IEEE-754)
Could be both signed and unsigned
Default
float
size is 64 bitPython automatically extends
float
when need bigger number
>>> data = 1.0
>>> data = +1.0
>>> data = -1.0
Floating-point numbers are not real numbers, so the result of 1.0/3.0
cannot be represented exactly without infinite precision. In the decimal
(base 10) number system, one-third is a repeating fraction, so it has an
infinite number of digits. Even simple non-repeating decimal numbers can
be a problem. One-tenth (0.1
) is obviously non-repeating, so we can
express it exactly with a finite number of digits. As it turns out, since
numbers within computers are stored in binary (base 2) form, even one-tenth
cannot be represented exactly with floating-point numbers.
When should you use integers and when should you use floating-point numbers? A good rule of thumb is this: use integers to count things and use floating-point numbers for quantities obtained from a measuring device. As examples, we can measure length with a ruler or a laser range finder; we can measure volume with a graduated cylinder or a flow meter; we can measure mass with a spring scale or triple-beam balance. In all of these cases, the accuracy of the measured quantity is limited by the accuracy of the measuring device and the competence of the person or system performing the measurement. Environmental factors such as temperature or air density can affect some measurements. In general, the degree of inexactness of such measured quantities is far greater than that of the floating-point values that represent them.
Despite their inexactness, floating-point numbers are used every day throughout the world to solve sophisticated scientific and engineering problems. The limitations of floating-point numbers are unavoidable since values with infinite characteristics cannot be represented in a finite way. Floating-point numbers provide a good trade-off of precision for practicality.
Note
Source [1]
3.2.1. Without Zero Notation
.1
- notation without leading zero (0.1)1.
- notation without trailing zero (1.0)Used by
numpy
Leading zero:
>>> data = .1
>>> print(data)
0.1
Trailing zero:
>>> data = 1.
>>> print(data)
1.0
3.2.2. Engineering Notation
The exponential is a number divisible by 3
Allows the numbers to explicitly match their corresponding SI prefixes
1e3
is equal to1000.0
(kilo)1e-3
is equal to0.001
(milli)
You can use both lower e
or uppercase letter E
:
>>> x = 1e3
>>> print(x)
1000.0
>>>
>>> x = 1E3
>>> print(x)
1000.0
Both negative and positive exponents are supported:
>>> x = 1e3
>>> print(x)
1000.0
>>>
>>> x = 1e-3
>>> print(x)
0.001
Both negative and positive numbers are supported:
>>> x = +1e3
>>> print(x)
1000.0
>>>
>>> x = -1e3
>>> print(x)
-1000.0
Engineering notation with prefixes:
>>> yocto = 1e-24 # 0.000000000000000000000001.0
>>> zepto = 1e-21 # 0.000000000000000000001.0
>>> atto = 1e-18 # 0.000000000000000001.0
>>> femto = 1e-15 # 0.000000000000001.0
>>> pico = 1e-12 # 0.000000000001.0
>>> nano = 1e-9 # 0.000000001.0
>>> micro = 1e-6 # 0.000001.0
>>> milli = 1e-3 # 0.001.0
>>> #
>>> kilo = 1e3 # 1000.0
>>> mega = 1e6 # 1000000.0
>>> giga = 1e9 # 1000000000.0
>>> tera = 1e12 # 1000000000000.0
>>> peta = 1e15 # 1000000000000000.0
>>> exa = 1e18 # 1000000000000000000.0
>>> zetta = 1e21 # 1000000000000000000000.0
>>> yotta = 1e24 # 1000000000000000000000000.0
3.2.3. Scientific notation
1.23e3
is equal to1230.0
1.23e-3
is equal to1.23e-3
>>> 1.23e3
1230.0
>>>
>>> 1.23e-3
0.00123
For numbers below 4 decimal places, Python will use notation automatically:
>>> 1.23e-4
0.000123
>>>
>>> 1.23e-5
1.23e-05
Both negative and positive numbers are supported:
>>> 1.23e3
1230.0
>>>
>>> -1.23e3
-1230.0
>>>
>>> 1.23e-3
0.00123
>>>
>>> -1.23e-3
-0.00123
3.2.4. Conversion
Builtin
float()
converts argument tofloat
Builtin float()
converts argument to float
>>> float(1)
1.0
>>>
>>> float('1.0')
1.0
>>> float('+1.0')
1.0
>>>
>>> float('-1.0')
-1.0
3.2.5. Thousand separator
Underscore (
_
) can be used as a thousand separator
>>> data = 1_000_000.0
>>> data = 0.000_0001
>>> data = 1_000_000.000_0001
3.2.6. Decimal Separator
1.0
- Decimal point1,0
- Decimal comma0٫1
- Arabic decimal separator (Left to right)More information: [2]
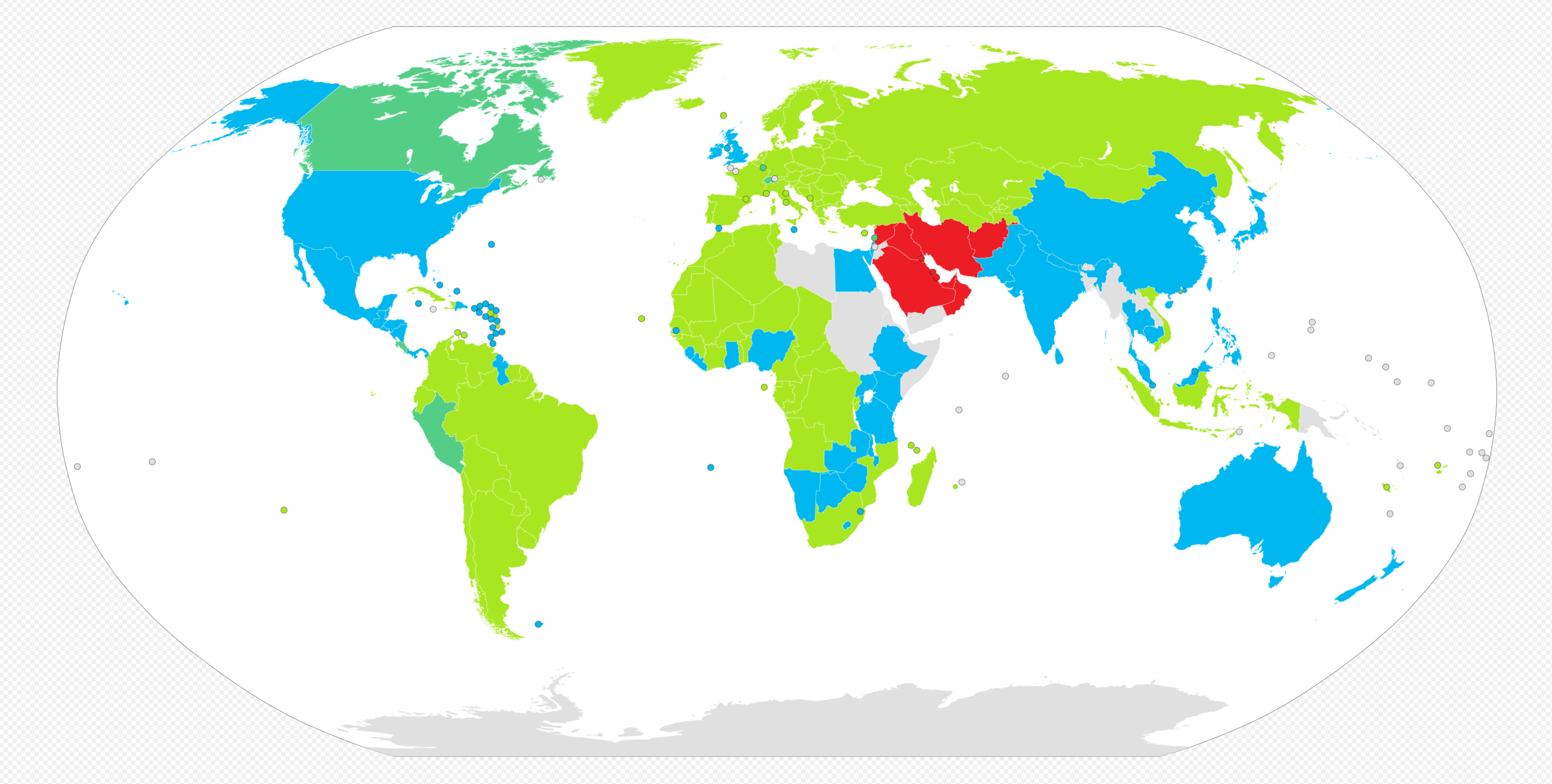
>>> data = 1.0
>>> type(data)
<class 'float'>
>>>
>>> data = 1,0
>>> type(data)
<class 'tuple'>
>>> float('1.0')
1.0
>>>
>>> float('1,0')
Traceback (most recent call last):
ValueError: could not convert string to float: '1,0'
3.2.7. Round Number
round(number, ndigits)
- Round a number to n decimal places
>>> pi = 3.14159265359
>>>
>>>
>>> round(pi, 4)
3.1416
>>>
>>> round(pi, 2)
3.14
>>>
>>> round(pi, 0)
3.0
>>>
>>> round(pi)
3
Rounding a number in string formatting:
>>> pi = 3.14159265359
>>>
>>>
>>> print(f'Pi number is {pi}')
Pi number is 3.14159265359
>>>
>>> print(f'Pi number is {pi:.4f}')
Pi number is 3.1416
>>>
>>> print(f'Pi number is {pi:.2f}')
Pi number is 3.14
>>>
>>> print(f'Pi number is {pi:.0f}')
Pi number is 3
3.2.8. Further Reading
Wikipedia. Decimal separator. Year: 2024. Retrieved: 2024-07-01. URL: https://en.wikipedia.org/wiki/Decimal_separator
3.2.9. References
3.2.10. Assignments
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Tax
# - Difficulty: easy
# - Lines: 1
# - Minutes: 2
# %% English
# 1. Price of the service is 123.00 Euro-cent
# 2. Define `result: float` with price in EUR
# 3. Run doctests - all must succeed
# %% Polish
# 1. Cena usługi wynosi 123.00 Euro-cent
# 2. Zdefiniuj `result: float` ceną w EUR
# 3. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert result is not Ellipsis, \
'Assign your result to variable `result`'
>>> assert type(result) is float, \
'Variable `result` has invalid type, should be float'
>>> pprint(result)
1.23
"""
CENT = 1
EUR = 100*CENT
PRICE = 123.00*CENT
# PRICE in EURO
# type: float
result = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Tax
# - Difficulty: easy
# - Lines: 4
# - Minutes: 3
# %% English
# 1. Cost of the service is 100.00 EUR
# 2. Calculate price in Polish Zloty PLN (PLN)
# 3. Calculate price in US Dollars (USD)
# 4. Calculate price in Australian Dollars (AUD)
# 5. Calculate price in Canadian Dollars (CAD)
# 3. Run doctests - all must succeed
# %% Polish
# 1. Cena usługi wynosi 100.00 EUR
# 2. Oblicz kwotę w polskich złotych (PLN)
# 3. Oblicz kwotę w dolarach amerykańskich (USD)
# 4. Oblicz kwotę w dolarach australijskich (AUD)
# 5. Oblicz kwotę w dolarach kanadyjskich (CAD)
# 3. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert result_a is not Ellipsis, \
'Assign your result to variable `result_a`'
>>> assert type(result_a) is float, \
'Variable `result_a` has invalid type, should be float'
>>> assert result_b is not Ellipsis, \
'Assign your result to variable `result_b`'
>>> assert type(result_b) is float, \
'Variable `result_b` has invalid type, should be float'
>>> assert result_c is not Ellipsis, \
'Assign your result to variable `result_c`'
>>> assert type(result_c) is float, \
'Variable `result_c` has invalid type, should be float'
>>> assert result_d is not Ellipsis, \
'Assign your result to variable `result_d`'
>>> assert type(result_d) is float, \
'Variable `result_d` has invalid type, should be float'
>>> result = round(result_a, 1)
>>> pprint(result)
435.0
>>> result = round(result_b, 1)
>>> pprint(result)
110.0
>>> result = round(result_c, 1)
>>> pprint(result)
166.0
>>> result = round(result_d, 1)
>>> pprint(result)
149.0
"""
EUR = 1
PLN = EUR / 4.35
USD = EUR / 1.10
AUD = EUR / 1.66
CAD = EUR / 1.49
PRICE = 100*EUR
# PRICE in Polish Zloty PLN (PLN)
# type: float
result_a = ...
# PRICE in US Dollars (USD)
# type: float
result_b = ...
# PRICE in Australian Dollars (AUD)
# type: float
result_c = ...
# PRICE in Canadian Dollars (CAD)
# type: float
result_d = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Tax
# - Difficulty: easy
# - Lines: 1
# - Minutes: 3
# %% English
# 1. Cost of the service is 1013.25 EUR net
# 2. Service has value added tax (VAT) rate of 21%
# 3. Calculate gross values (with tax)
# 4. Run doctests - all must succeed
# %% Polish
# 1. Cena usługi wynosi 1013.25 EUR netto
# 2. Usługa objęta jest 21% stawką VAT
# 3. Oblicz cenę brutto (z podatkiem)
# 4. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert result is not Ellipsis, \
'Assign your result to variable `result`'
>>> assert type(result) is float, \
'Variable `result` has invalid type, should be float'
>>> result = round(result, 2)
>>> pprint(result)
1226.03
"""
EUR = 1.00
VAT = 1.21
PRICE = 1013.25*EUR
# Gross value for 1013.25 EUR net price
# type: float
result = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Tax
# - Difficulty: easy
# - Lines: 1
# - Minutes: 3
# %% English
# 1. Cost of the service is 1013.25 EUR net
# 2. Service has value added tax (VAT) rate of 21%
# 3. Calculate VAT tax
# 4. Run doctests - all must succeed
# %% Polish
# 1. Cena usługi wynosi 1013.25 EUR netto
# 2. Usługa objęta jest 21% stawką VAT
# 3. Oblicz wartości podatku VAT
# 4. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert result is not Ellipsis, \
'Assign your result to variable `result`'
>>> assert type(result) is float, \
'Variable `result` has invalid type, should be float'
>>> result = round(result, 2)
>>> pprint(result)
212.78
"""
EUR = 1.00
VAT = 0.21
PRICE = 1013.25*EUR
# VAT tax for 1013.25 EUR net price
# type: float
result = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Altitude
# - Difficulty: easy
# - Lines: 2
# - Minutes: 3
# %% English
# 1. Plane altitude is 10 km
# 2. Convert to feet (ft) in imperial system (US)
# 3. Convert to meters (m) in metric system (SI)
# 4. Run doctests - all must succeed
# %% Polish
# 1. Wysokość lotu samolotem wynosi 10 km
# 2. Przelicz je na stopy (ft) w systemie imperialnym (US)
# 3. Przelicz je na metry (m) w systemie metrycznym (układ SI)
# 4. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert altitude_m is not Ellipsis, \
'Assign your result to variable `altitude_m`'
>>> assert altitude_ft is not Ellipsis, \
'Assign your result to variable `altitude_ft`'
>>> assert type(altitude_m) is float, \
'Variable `altitude_m` has invalid type, should be float'
>>> assert type(altitude_ft) is float, \
'Variable `altitude_ft` has invalid type, should be float'
>>> result = round(altitude_m, 1)
>>> pprint(result)
10000.0
>>> result = round(altitude_ft, 1)
>>> pprint(result)
32808.4
"""
m = 1
km = 1000 * m
ft = 0.3048 * m
ALTITUDE = 10*km
# ALTITUDE in meters
# type: float
altitude_m = ...
# ALTITUDE in feet
# type: float
altitude_ft = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Volume
# - Difficulty: easy
# - Lines: 2
# - Minutes: 3
# %% English
# 1. Bottle volume is 500 mililiter
# 2. Convert to fluid unces (floz) in imperial system (US)
# 3. Convert to liters (liter) in metric system (SI)
# 4. Run doctests - all must succeed
# %% Polish
# 1. Objętość butelki wynosi 500 mililitrów
# 2. Przelicz je na uncje płynu (floz) w systemie imperialnym (US)
# 3. Przelicz je na litry (liter) w systemie metrycznym (układ SI)
# 4. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert volume_floz is not Ellipsis, \
'Assign your result to variable `volume_floz`'
>>> assert volume_l is not Ellipsis, \
'Assign your result to variable `volume_l`'
>>> assert type(volume_floz) is float, \
'Variable `volume_floz` has invalid type, should be float'
>>> assert type(volume_l) is float, \
'Variable `volume_l` has invalid type, should be float'
>>> result = round(volume_floz, 1)
>>> pprint(result)
16.9
>>> result = round(volume_l, 1)
>>> pprint(result)
0.5
"""
liter = 1
milliliter = 0.001 * liter
floz = 0.02957344 * liter
VOLUME = 500 * milliliter
# VOLUME in fluid ounces
# type: float
volume_floz = ...
# VOLUME in liters
# type: float
volume_l = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Round
# - Difficulty: easy
# - Lines: 4
# - Minutes: 5
# %% English
# 1. Euler's number is 2.71828
# 2. Round number using `round()` funtion
# 3. Run doctests - all must succeed
# %% Polish
# 1. Liczba Eulra to 2.71828
# 2. Zaokrąglij liczbę wykorzystując funkcję `round()`
# 3. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert a is not Ellipsis, \
'Assign your result to variable `a`'
>>> assert b is not Ellipsis, \
'Assign your result to variable `b`'
>>> assert c is not Ellipsis, \
'Assign your result to variable `c`'
>>> assert d is not Ellipsis, \
'Assign your result to variable `d`'
>>> assert e is not Ellipsis, \
'Assign your result to variable `e`'
>>> assert type(a) is float, \
'Variable `a` has invalid type, should be float'
>>> assert type(b) is float, \
'Variable `b` has invalid type, should be float'
>>> assert type(c) is float, \
'Variable `c` has invalid type, should be float'
>>> assert type(d) is float, \
'Variable `d` has invalid type, should be float'
>>> assert type(e) is int, \
'Variable `e` has invalid type, should be int'
>>> pprint(a)
2.718
>>> pprint(b)
2.72
>>> pprint(c)
2.7
>>> pprint(d)
3.0
>>> pprint(e)
3
"""
EULER = 2.71828
# Round Euler's number to 3 decimal places
# Use `round()`
# type: float
a = ...
# Round Euler's number to 2 decimal places
# Use `round()`
# type: float
b = ...
# Round Euler's number to 1 decimal places
# Use `round()`
# type: float
c = ...
# Round Euler's number to 0 decimal places
# Use `round()`
# type: float
d = ...
# Round Euler's number to nearest integer
# Use `round()`
# type: int
e = ...
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Round
# - Difficulty: easy
# - Lines: 4
# - Minutes: 5
# %% English
# 1. Euler's number is 2.71828
# 2. Round number using f-string formatting,
# example: f'{number:.2f}'
# 3. Run doctests - all must succeed
# %% Polish
# 1. Liczba Eulra to 2.71828
# 2. Zaokrąglij liczbę wykorzystując formatowanie f-string,
# przykład: f'{liczba:.2f}'
# 3. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert a is not Ellipsis, \
'Assign your result to variable `a`'
>>> assert b is not Ellipsis, \
'Assign your result to variable `b`'
>>> assert c is not Ellipsis, \
'Assign your result to variable `c`'
>>> assert d is not Ellipsis, \
'Assign your result to variable `d`'
>>> assert type(a) is str, \
'Variable `a` has invalid type, should be str'
>>> assert type(b) is str, \
'Variable `b` has invalid type, should be str'
>>> assert type(c) is str, \
'Variable `c` has invalid type, should be str'
>>> assert type(d) is str, \
'Variable `d` has invalid type, should be str'
>>> pprint(a)
'Result: 2.718'
>>> pprint(b)
'Result: 2.72'
>>> pprint(c)
'Result: 2.7'
>>> pprint(d)
'Result: 3'
"""
EULER = 2.71828
# Round Euler's number to 3 decimal places
# Round number using f-string formatting
# type: str
a = f"Result: {EULER}"
# Round Euler's number to 2 decimal places
# Round number using f-string formatting
# type: str
b = f"Result: {EULER}"
# Round Euler's number to 1 decimal places
# Round number using f-string formatting
# type: str
c = f"Result: {EULER}"
# Round Euler's number to 0 decimal places
# Round number using f-string formatting
# type: str
d = f"Result: {EULER}"
# %% License
# - Copyright 2025, Matt Harasymczuk <matt@python3.info>
# - This code can be used only for learning by humans
# - This code cannot be used for teaching others
# - This code cannot be used for teaching LLMs and AI algorithms
# - This code cannot be used in commercial or proprietary products
# - This code cannot be distributed in any form
# - This code cannot be changed in any form outside of training course
# - This code cannot have its license changed
# - If you use this code in your product, you must open-source it under GPLv2
# - Exception can be granted only by the author
# %% Run
# - PyCharm: right-click in the editor and `Run Doctest in ...`
# - PyCharm: keyboard shortcut `Control + Shift + F10`
# - Terminal: `python -m doctest -v myfile.py`
# %% About
# - Name: Numeric Float Velocity
# - Difficulty: easy
# - Lines: 2
# - Minutes: 3
# %% English
# 1. Speed limit is 75 MPH
# 2. Convert to miles per hour (mph) in imperial system (US)
# 3. Convert to kilometers per hour (kph) in metric system (SI)
# 4. Speed limit print in KPH (km/h)
# 5. Run doctests - all must succeed
# %% Polish
# 1. Ograniczenie prędkości wynosi 75 MPH
# 2. Przelicz je na mile na godzinę (mph) w systemie imperialnym (US)
# 3. Przelicz je na kilometry na godzinę (kph) w systie metrycznym (układ SI)
# 4. Ograniczenie prędkości wypisz w KPH (km/h)
# 5. Uruchom doctesty - wszystkie muszą się powieść
# %% Tests
"""
>>> import sys; sys.tracebacklimit = 0
>>> assert sys.version_info >= (3, 9), \
'Python 3.9+ required'
>>> from pprint import pprint
>>> assert speed_limit_mph is not Ellipsis, \
'Assign your result to variable `speed_limit_mph`'
>>> assert speed_limit_kph is not Ellipsis, \
'Assign your result to variable `speed_limit_kph`'
>>> assert type(speed_limit_mph) is float, \
'Variable `speed_limit_mph` has invalid type, should be float'
>>> assert type(speed_limit_kph) is float, \
'Variable `speed_limit_kph` has invalid type, should be float'
>>> result = round(speed_limit_mph, 1)
>>> pprint(result)
75.0
>>> result = round(speed_limit_kph, 1)
>>> pprint(result)
120.7
"""
SECOND = 1
MINUTE = 60 * SECOND
HOUR = 60 * MINUTE
m = 1
km = 1000 * m
mi = 1609.344 * m
kph = km / HOUR
mph = mi / HOUR
SPEED_LIMIT = 75 * mph
# SPEED_LIMIT in miles per hour
# type: float
speed_limit_mph = ...
# SPEED_LIMIT in kilometers per hour
# type: float
speed_limit_kph = ...