4.6. Inheritance Composition
4.6.1. Diagram
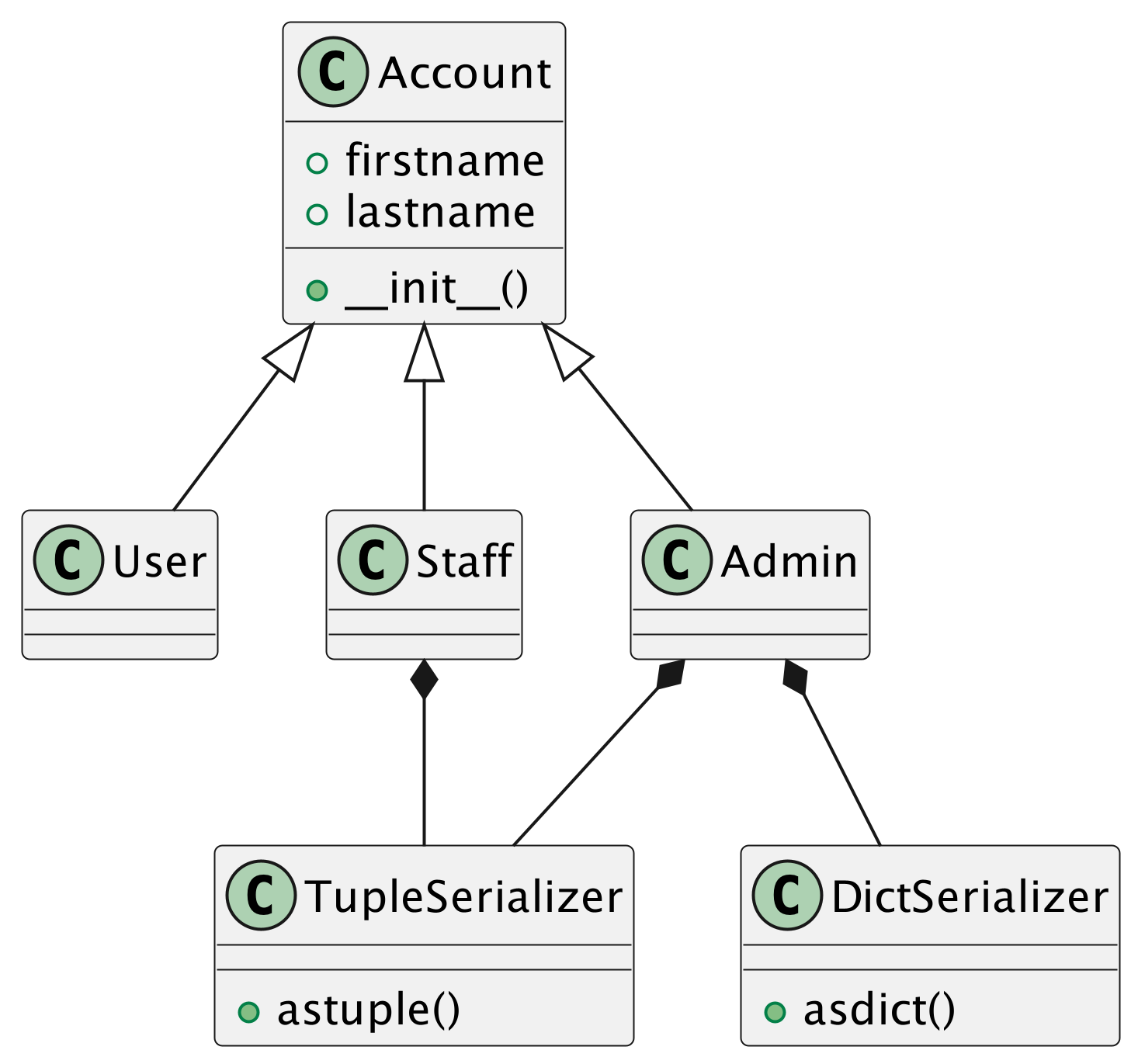
4.6.2. Code
class TupleSerializer:
def astuple(self, instance):
data = [v for v in vars(instance).values()]
return tuple(data)
class DictSerializer:
def asdict(self, instance):
data = {k: v for k, v in vars(instance).items()
if not k.startswith('_')}
return dict(data)
class Account:
tuple_serializer: TupleSerializer | None
dict_serializer: DictSerializer | None
def __init__(self, firstname, lastname):
self.firstname = firstname
self.lastname = lastname
def astuple(self):
if self.tuple_serializer is None:
clsname = self.__class__.__name__
error = f"'{clsname}' object has no attribute 'astuple'"
raise AttributeError(error)
return self.tuple_serializer.astuple(self)
def asdict(self):
if self.dict_serializer is None:
clsname = self.__class__.__name__
error = f"'{clsname}' object has no attribute 'asdict'"
raise AttributeError(error)
return self.dict_serializer.asdict(self)
class User(Account):
tuple_serializer = None
dict_serializer = None
class Staff(Account):
tuple_serializer = TupleSerializer()
dict_serializer = None
class Admin(Account):
tuple_serializer = TupleSerializer()
dict_serializer = DictSerializer()
4.6.3. Usage
>>> mark = User('Mark', 'Watney')
>>>
>>> mark.astuple()
Traceback (most recent call last):
AttributeError: 'User' object has no attribute 'astuple'
>>>
>>> mark.asdict()
Traceback (most recent call last):
AttributeError: 'User' object has no attribute 'asdict'
>>> rick = Staff('Rick', 'Martinez')
>>>
>>> rick.astuple()
('Rick', 'Martinez')
>>>
>>> rick.asdict()
Traceback (most recent call last):
AttributeError: 'Staff' object has no attribute 'asdict'
>>> melissa = Admin('Melissa', 'Lewis')
>>>
>>> melissa.astuple()
('Melissa', 'Lewis')
>>>
>>> melissa.asdict()
{'firstname': 'Melissa', 'lastname': 'Lewis'}
4.6.4. Extensibility
>>> class MyTupleSerializer(TupleSerializer):
... def astuple(self, instance):
... data = super().astuple(instance)
... print('do something extra')
... return tuple(data)
>>> mark = User('Mark', 'Watney', tuple_serializer=MyTupleSerializer)
>>>
>>> mark.astuple()
do something extra
('Mark', 'Watney')
4.6.5. Use Case - 0x01
>>> class UserPermissions:
... pass
>>>
>>> class AdminPermissions:
... pass
>>>
>>> class MyAccount:
... permissions: UserPermissions | AdminPermissions
...
... def __init__(self, permissions=UserPermissions()):
... self.permissions = permissions
4.6.6. Use Case - 0x02
SetUp:
>>> import json
>>> from datetime import date
Naive example:
>>> data = {
... 'firstname': 'Mark',
... 'lastname': 'Watney',
... }
>>>
>>> json.dumps(data)
'{"firstname": "Mark", "lastname": "Watney"}'
Let's add a birthdate:
>>> data = {
... 'firstname': 'Mark',
... 'lastname': 'Watney',
... 'birthdate': date(1969, 7, 21),
... }
>>>
>>> json.dumps(data)
Traceback (most recent call last):
TypeError: Object of type date is not JSON serializable
If you want to use your better version of encoder (for example which
can encode date
object. You can create a class which inherits
from default json.JSONEncoder
and overwrite .default()
method.
Here's the solution:
>>> class MyEncoder(json.JSONEncoder):
... def default(self, obj):
... if isinstance(obj, date):
... return obj.isoformat()
>>>
>>>
>>> data = {
... 'firstname': 'Mark',
... 'lastname': 'Watney',
... 'birthdate': date(1969, 7, 21),
... }
>>>
>>> json.dumps(data, cls=MyEncoder)
'{"firstname": "Mark", "lastname": "Watney", "birthdate": "1969-07-21"}'