16.11. OOP UML
16.11.1. Value
Type + instance = Value
Type instances create Values:
>>> class str:
... ...
...
>>> 'Mark'
>>> 'Watney'
16.11.2. Scalars
Value + Identifier = Scalar
>>> firstname = 'Mark'
>>> lastname = 'Watney'
16.11.3. Structure
Scalar + Relation = Structure
>>> user = ['Mark', 'Watney']
16.11.4. Data
Structures + Meaning = Data
>>> user = {
... 'firstname': 'Mark',
... 'lastname': 'Watney',
... }
16.11.5. Information
Data + Behavior = Information
>>> class User:
... firstname: str
... lastname: str
...
... def __init__(self, firstname, lastname):
... self.firstname = firstname
... self.lastname = lastname
... self._authenticated = False
...
... def login(self):
... self._authenticated = True
...
... def logout(self):
... self._authenticated = False
>>>
>>>
>>> user = User('Mark', 'Watney')
>>>
>>> user.login()
>>> user.logout()
16.11.6. Application
Information + Business Logic = Application
>>> class User:
... firstname: str
... lastname: str
...
... def __init__(self, firstname, lastname):
... self.firstname = firstname
... self.lastname = lastname
... self._authenticated = False
...
... def login(self, username, password):
... if not (username == 'mwatney' and password == 'Ares3'):
... raise PermissionError('Invalid username and/or password')
... self._authenticated = True
...
... def logout(self):
... self._authenticated = False
>>>
>>>
>>> user = User('Mark', 'Watney')
>>>
>>> user.login('mwatney', 'Ares3')
>>> user.logout()
16.11.7. UML Class Diagram
Unified Modeling Language
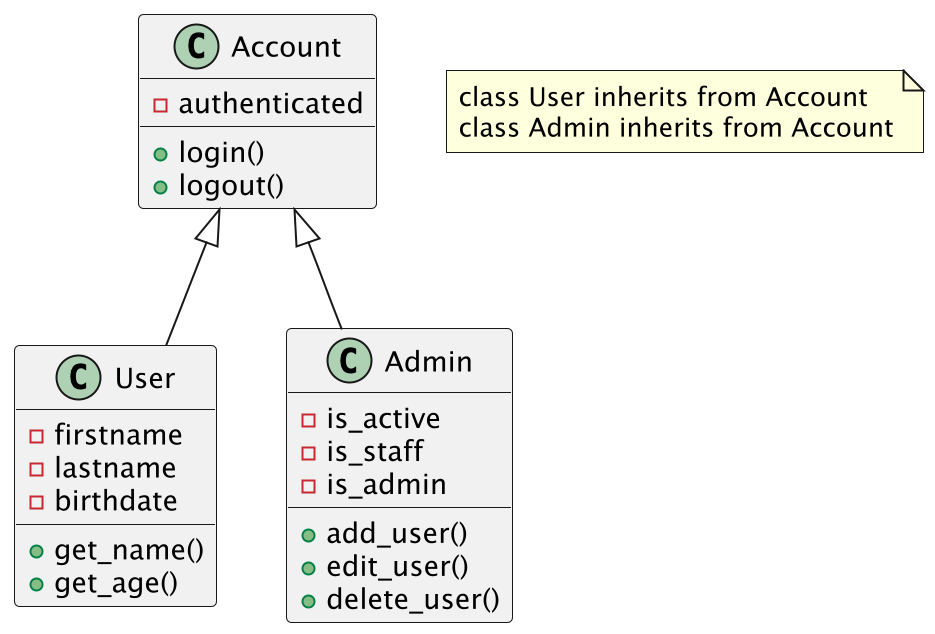
>>> class Account:
... def __init__(self):
... self._authenticated = False
...
... def login(self):
... self._authenticated = True
...
... def logout(self):
... self._authenticated = False
>>>
>>>
>>> class User(Account):
... def __init__(self, firstname, lastname, birthdate):
... self.firstname = firstname
... self.lastname = lastname
... self.birthdate = birthdate
...
... def get_name(self):
... return f'{self.firstname} {self.lastname}'
...
... def get_age(self):
... return ...
>>>
>>>
>>> class Admin(Account):
... def __init__(self):
... self.is_active = True
... self.is_staff = True
... self.is_admin = True
...
... def add_user(self):
... ...
...
... def edit_user(self):
... ...
...
... def delete_user(self):
... ...
16.11.8. UML Sequence Diagram
Unified Modeling Language
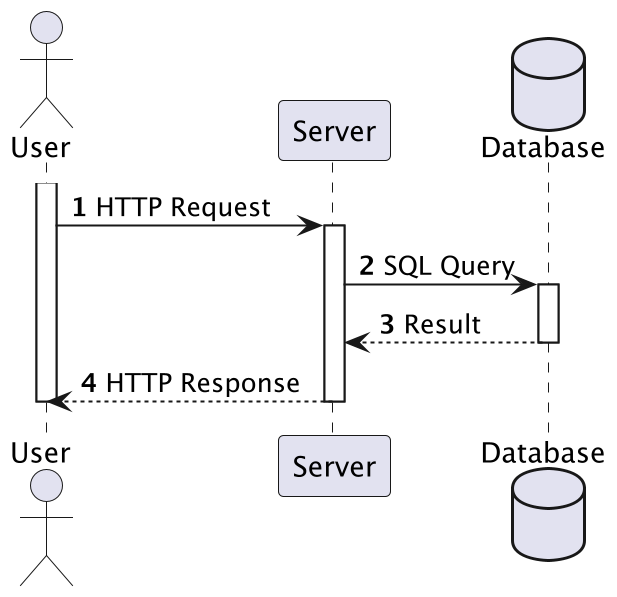
16.11.9. Use Case - 1
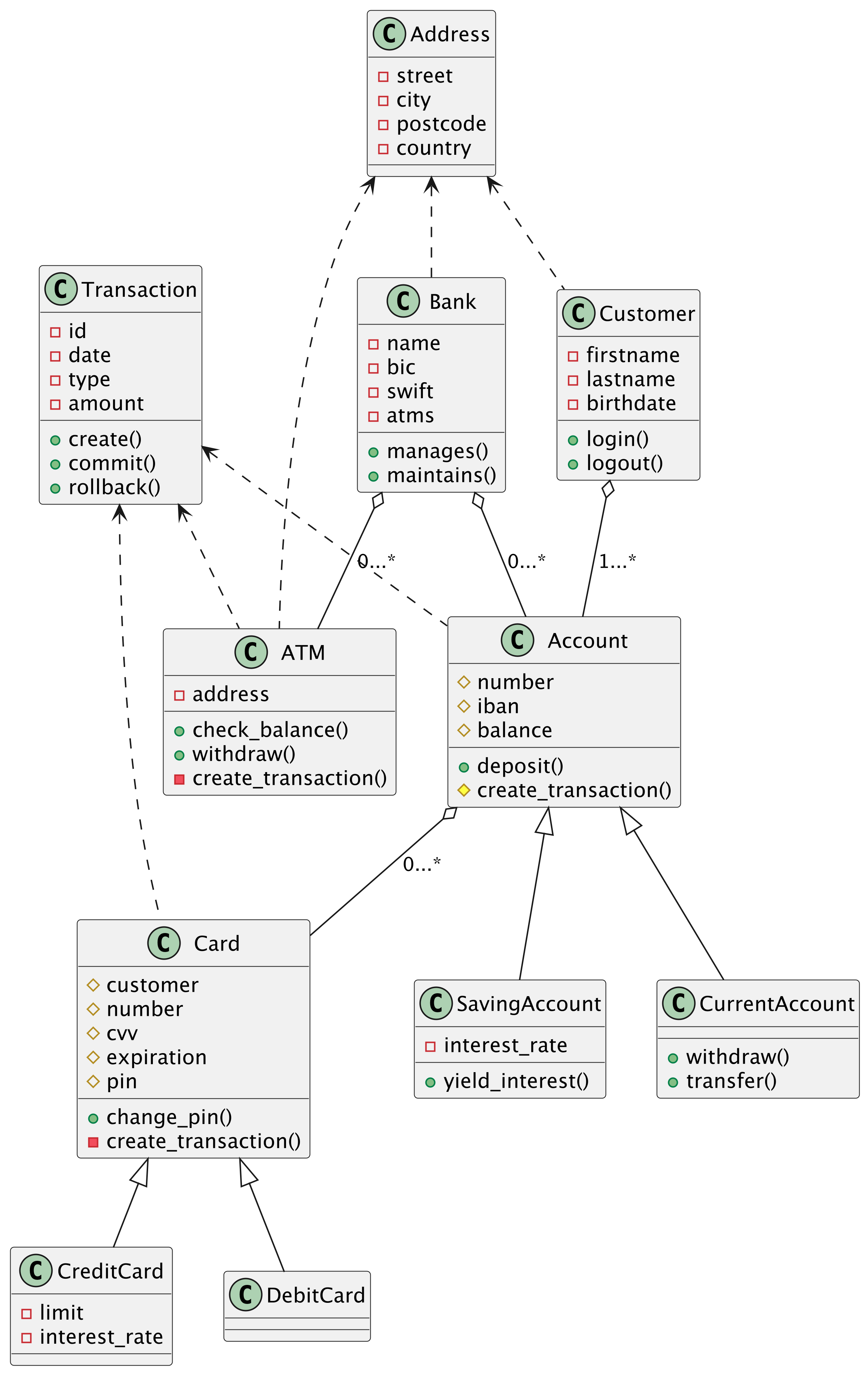
from decimal import Decimal
from datetime import date, datetime
class Address:
street: str
city: str
postcode: str
country: str
class Bank:
name: str
bic: str
swift: str
address: Address
def manages(self): ...
def maintains(self): ...
class Customer:
firstname: str
lastname: str
birthdate: date
address: Address
def login(self): ...
def logout(self): ...
class Card:
customer: Customer
number: int
cvv: int
expiration: date
pin: int
def change_pin(self): ...
def create_transaction(self): ...
class CreditCard(Card):
limit: Decimal
interest_rate: float
class DebitCard(Card):
pass
class ATM:
bank: Bank
address: Address
def check_balance(self): ...
def withdraw(self): ...
def create_transaction(self): ...
class Transaction:
id: str
date: datetime
type: str
amount: Decimal
def create(self): ...
def commit(self): ...
def rollback(self): ...
class Account:
number: int
iban: str
balance: Decimal
def deposit(self): ...
def create_transaction(self): ...
class CurrentAccount:
def withdraw(self): ...
def transfer(self): ...
class SavingAccount:
interest_rate: float
def yield_interest(self): ...