5.3. OOP Identity
id()
will change every time you execute scriptid()
returns an integer which is guaranteed to be unique and constant for object during its lifetimeTwo objects with non-overlapping lifetimes may have the same
id()
valueIn CPython it's also the memory address of the corresponding C object
Since Python 3.8 - Compiler produces a
SyntaxWarning
when identity checks (is
andis not
) are used with certain types of literals (e.g.str
,int
). These can often work by accident in CPython, but are not guaranteed by the language spec. The warning advises users to use equality tests (==
and!=
) instead.is
checks for object identityis
comparesid()
output for both objectsCPython: compares the memory address a object resides in
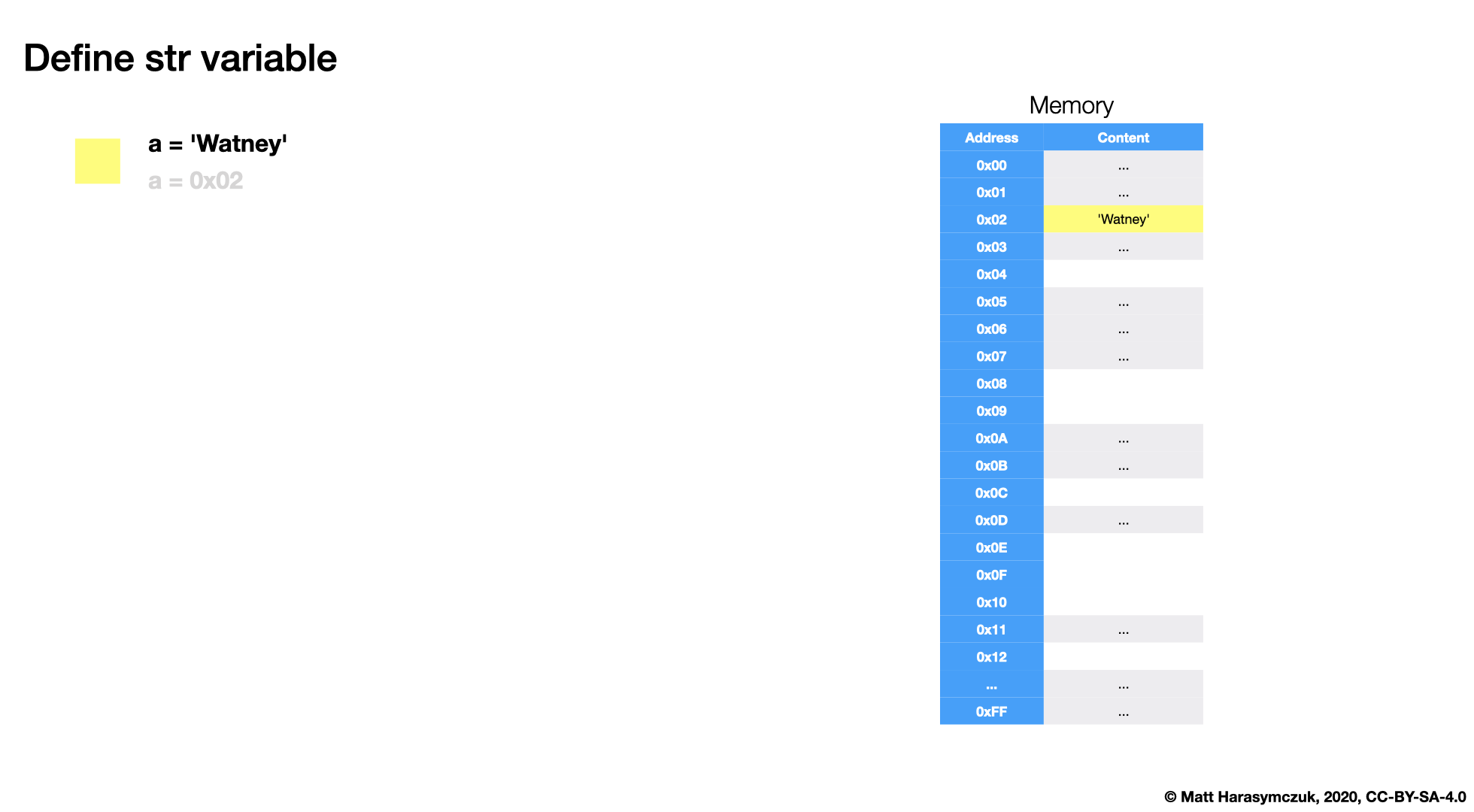

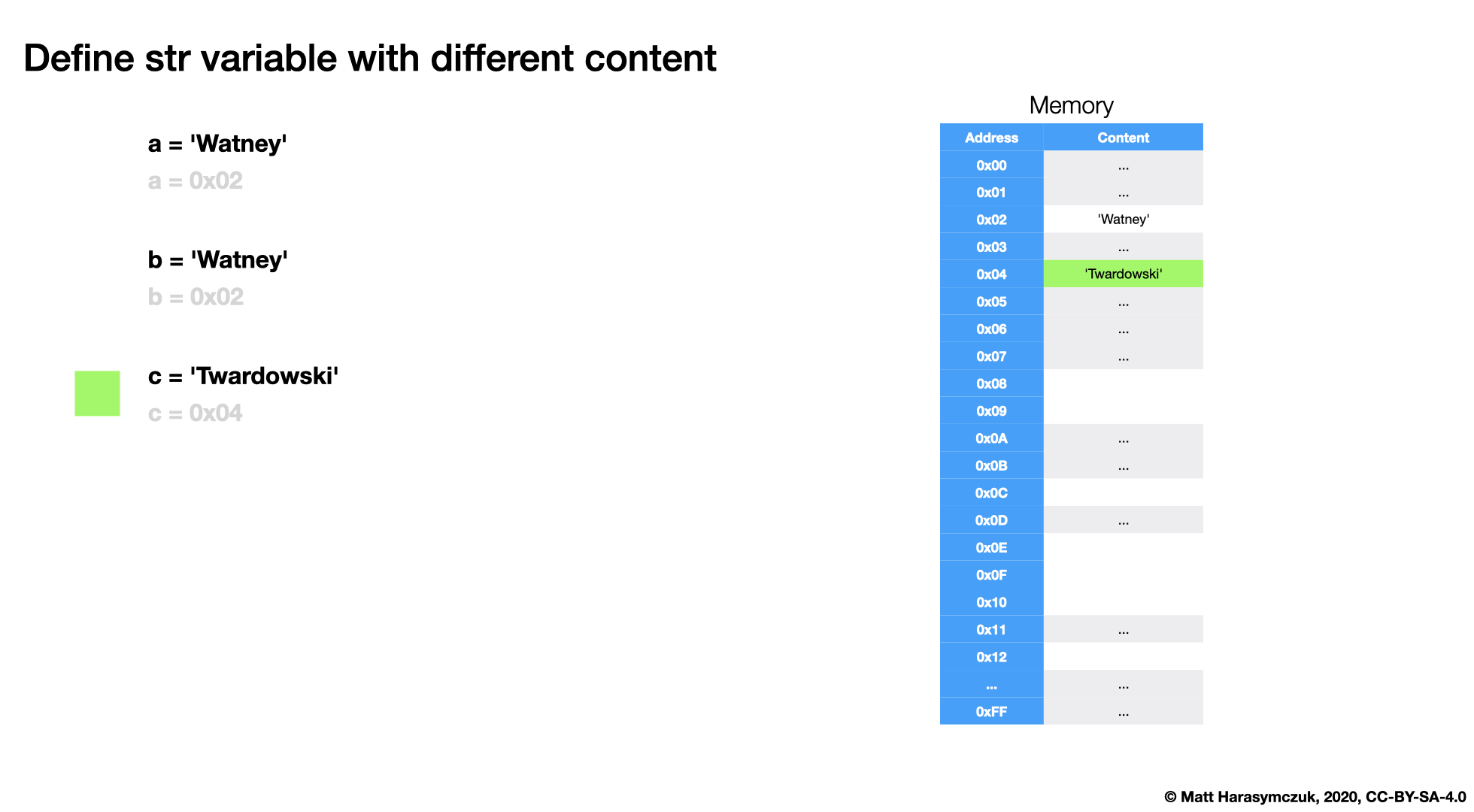
5.3.1. Builtin id()
id(obj) -> int
- Functionid()
returns an integerGuaranteed to be unique and constant for object during its lifetime
Will change every time you execute script
In CPython
id()
shows the memory address of an objectReturned value of
id()
will change every time you execute script
>>> class User:
... pass
>>>
>>>
>>> mark = User()
>>>
>>> mark
<__main__.User at 0x12068b8f0>
In CPython id()
shows the memory address of an object:
>>> id(mark)
4838701296
Usually memory address is shown in hexadecimal format:
>>> hex(4838701296)
'0x12068b8f0'
5.3.2. None Type Identity
NoneType object is a singleton
It always has the same identity (during one run)
>>> x = None
>>>
>>> x == None
True
>>>
>>> x is None
True
Rationale:
>>> id(x)
4351779640
>>>
>>> id(None)
4351779640
It always has the same identity (during one run):
>>> id(None)
4351779640
>>>
>>> id(None)
4351779640
>>>
>>> id(None)
4351779640
>>> x = None
>>> id(x)
4351779640
>>>
>>> x = [None, None, None]
>>> id(x[0])
4351779640
Performance:
>>> x = None
>>>
>>> # doctest: +SKIP
... %%timeit -r1000 -n1000
... x is None
...
10.9 ns ± 4.15 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
10.8 ns ± 6.06 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
9.98 ns ± 4.66 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
9.57 ns ± 3.63 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
10.4 ns ± 4.7 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
>>> x = None
>>>
>>> # doctest: +SKIP
... %%timeit -r1000 -n1000
... x == None
...
15.8 ns ± 3.76 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
15.4 ns ± 3.59 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
15.3 ns ± 4.04 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
15.7 ns ± 4.4 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
15.4 ns ± 4.12 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
Date: 2024-12-12
Python: 3.13.1
IPython: 8.30.0
System: macOS 15.1.1
Computer: MacBook M3 Max
CPU: 16 cores (12 performance and 4 efficiency) / 3nm
RAM: 128 GB RAM LPDDR5
Why:
>>> x.__eq__(None)
True
>>>
>>> None.__eq__(x)
True
>>>
>>> object.__eq__(x, None)
True
>>> x is None # and finally, this will be executed
True
5.3.3. Bool Type Identity
Bool object is a singleton
It always has the same identity (during one run)
>>> x = True
>>>
>>> x == True
True
>>>
>>> x is True
True
Rationale:
>>> id(x)
4379755984
>>>
>>> id(True)
4379755984
Performance:
>>> x = True
>>>
>>> # doctest: +SKIP
... %%timeit -r1000 -n1000
... x is True
...
9.26 ns ± 7.09 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
9.9 ns ± 3.49 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
10.3 ns ± 5.66 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
10.7 ns ± 4.19 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
10.6 ns ± 4.76 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
>>> x = True
>>>
>>> # doctest: +SKIP
... %%timeit -r1000 -n1000
... x == True
...
15.3 ns ± 5.38 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
15.6 ns ± 3.88 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
15.3 ns ± 8.11 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
13.9 ns ± 1.99 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
13.7 ns ± 4.74 ns per loop (mean ± std. dev. of 1000 runs, 1,000 loops each)
Date: 2024-12-12
Python: 3.13.1
IPython: 8.30.0
System: macOS 15.1.1
Computer: MacBook M3 Max
CPU: 16 cores (12 performance and 4 efficiency) / 3nm
RAM: 128 GB RAM LPDDR5
5.3.4. Int Type Identity
SyntaxWarning: "is" with 'int' literal. Did you mean "=="?
Integer interning (caching) for values from
-5
to256
-5
to256
are interned (cached) and have the sameid()
value-5
to256
are singletons-5
to256
are preallocated in memorythey are never deleted from memory (during one run)
>>> x = 1
>>>
>>> x == 1
True
>>>
>>> x is 1 # SyntaxWarning: "is" with 'int' literal. Did you mean "=="?
True
>>> x = 256
>>>
>>> x == 256
True
>>>
>>> x is 256 # SyntaxWarning: "is" with 'int' literal. Did you mean "=="?
True
>>> x = 257
>>> x == 257
True
>>> x is 257 # SyntaxWarning: "is" with 'int' literal. Did you mean "=="?
False
>>> x = -5
>>> x == -5
True
>>> x is -5 # SyntaxWarning: "is" with 'int' literal. Did you mean "=="?
True
>>> x = -6
>>> x == -6
True
>>> x is -6 # SyntaxWarning: "is" with 'int' literal. Did you mean "=="?
False
Rationale:
>>> data = ['a', 'b', 'c', 'd']
>>>
>>> data[0]
'a'
>>>
>>> data[1:3]
['b', 'c']
>>>
>>> data[slice(1,3)]
['b', 'c']
5.3.5. Float Type Identity
SyntaxWarning: "is" with 'float' literal. Did you mean "=="?
Floats are never cached
>>> x = 1.23
>>>
>>> x == 1.23
True
>>>
>>> x is 1.23 # SyntaxWarning: "is" with 'float' literal. Did you mean "=="?
False
5.3.6. String Types Identity
Strings are interned only if they do not contains special characters
SyntaxWarning: "is" with 'float' literal. Did you mean "=="?
>>> x = 'Czesc'
>>>
>>> x == 'Czesc'
True
>>>
>>> x is 'Czesc'
True
>>> x = 'Cześć'
>>>
>>> x == 'Cześć' # SyntaxWarning: "is" with 'float' literal. Did you mean "=="?
True
>>>
>>> x is 'Cześć'
False
5.3.7. Sequences Identity
>>> x = (1, 2, 3)
>>>
>>> x == (1, 2, 3)
True
>>>
>>> x is (1, 2, 3) # SyntaxWarning: "is" with 'tuple' literal. Did you mean "=="?
False
>>> x = [1, 2, 3]
>>>
>>> x == [1, 2, 3]
True
>>>
>>> x is [1, 2, 3]
False
>>> x = {1, 2, 3}
>>>
>>> x == {1, 2, 3}
True
>>>
>>> x is {1, 2, 3}
False
5.3.8. Mappings Identity
>>> x = {'a':1, 'b':2, 'c':3}
>>>
>>> x == {'a':1, 'b':2, 'c':3}
True
>>>
>>> x is {'a':1, 'b':2, 'c':3}
False
5.3.9. Collections Identity
Increment add with lists:
>>> data = [1, 2, 3]
>>> hex(id(data))
'0x106b4ab40'
>>>
>>> data += [4, 5, 6]
>>> hex(id(data))
'0x106b4ab40'
>>>
>>> data
[1, 2, 3, 4, 5, 6]
Increment add with tuples:
>>> data = (1, 2, 3)
>>> hex(id(data))
'0x10685e0c0'
>>>
>>> data += (4, 5, 6)
>>> hex(id(data))
'0x106b4cf40'
>>>
>>> data
(1, 2, 3, 4, 5, 6)
Tuple identities:
>>> (1,2,3) is (1,2,3) # SyntaxWarning: "is" with 'tuple' literal. Did you mean "=="?
True
>>> a = (1, 2, 3)
>>> b = (1, 2, 3)
>>>
>>> a is b # SyntaxWarning: "is" with 'tuple' literal. Did you mean "=="?
False
>>> a=(1,2,3); b=(1,2,3)
>>>
>>> a is b # SyntaxWarning: "is" with 'tuple' literal. Did you mean "=="?
True
Why:
>>> id(a)
4411490432
>>>
>>> id(b)
4421110208
5.3.10. Object Identity
>>> class User:
... pass
>>>
>>>
>>> x = User()
>>>
>>> x == User()
False
>>>
>>> x is User()
False
5.3.11. Class Identity
Class object is a singleton
It always has the same identity (during one run)
>>> class User:
... pass
>>>
>>>
>>> x = User()
>>>
>>> type(x) == User
True
>>>
>>> type(x) is User
True
5.3.12. Type Identity
Type object is a singleton
>>> class User:
... pass
>>>
>>>
>>> cls = User
>>>
>>> cls == User
True
>>>
>>> cls is User
True